Overview
Insurelytics is a desktop client management application for insurance agents. Insurelytics facilitates the tracking of client and policies, and also generate statistical analytics to provide insights for the agent. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 85 kLoC.
Summary of contributions
-
Major enhancement: Added Bin Feature
-
What it does: Added a bin to store all deleted items. When deleting either persons or policies, instead of being removed permanently, deleted items are shifted to the bin instead. Just like a recycling bin on a typical computer, items inside the bin can be restored as long as they have not exceeded their expiry date.
-
Justification: This allows for the user to restore persons or policies that could have been wrongly deleted in previous sessions. Additionally, this is also useful in the case when the user wants to restore an item that was deleted at the start of the session, where it is too troublesome to undo cleanly.
-
Highlights:
-
The bin is persistent across different sessions. Its items are saved into a json file, as with persons and policies.
-
Once the deleted items have exceeded their expiry date, they will be automatically deleted on restart of the application.
-
The amount of time an item stays in the bin before it is deleted can be set by the user as a user setting, through the command
binitemexpiry
. This is a global setting and applies for all items in the bin.
-
-
-
Minor enhancement:
-
Redesigned User Interface to give a cleaner, sleek look. [Pull Request #209]
-
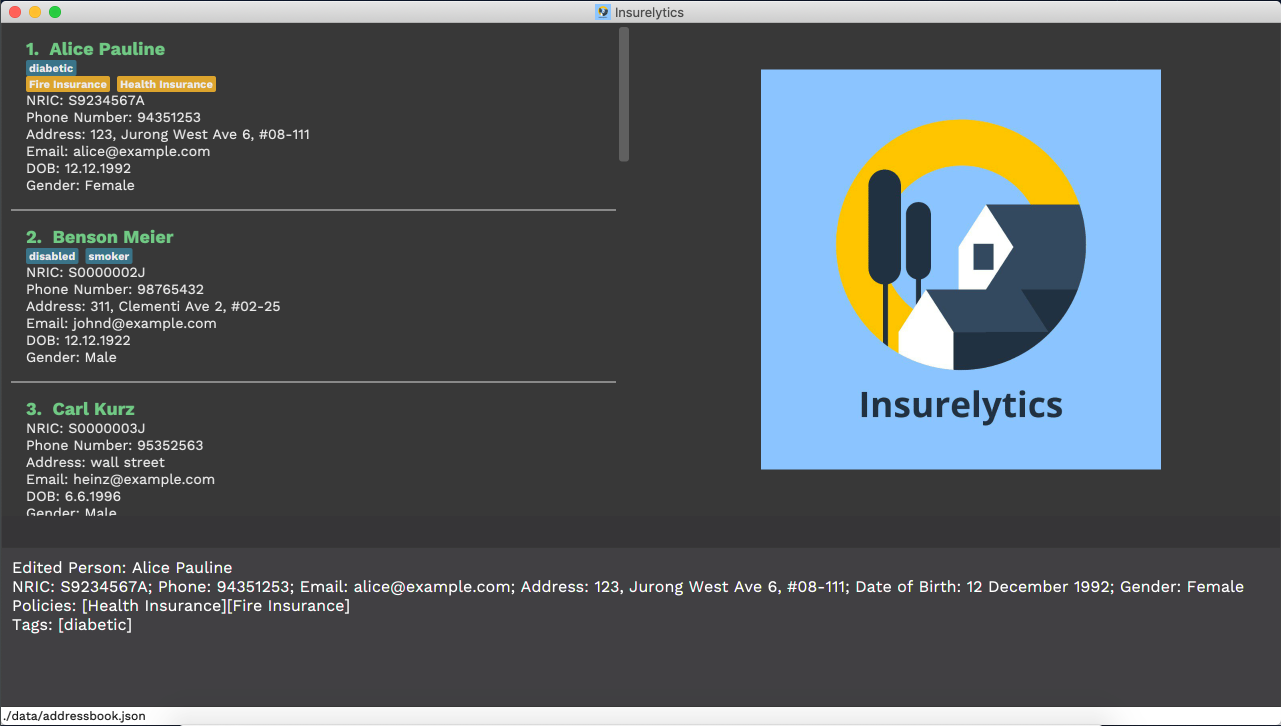
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Deleting a person : delete
Deletes the specified person from the list of people.
Format: delete INDEX
Expired items are removed on restart of application. |
Examples:
Delete the 2nd person in the list of people.
# input commands
>>> listpeople
>>> delete 2
Expected output:
Deleted Person: Benson Meier
NRIC: S0000002J; Phone: 98765432; Email: johnd@example.com; Address: 311, Clementi Ave 2, #02-25; Date of Birth: 12 December 1922; Gender: Male
Policies: [Life Insurance]
Tags: [smoker][disabled]
Delete the 1st person in the results of the find
command.
# input commands
>>> find carl
>>> delete 1
Expected output:
Deleted Person: Carl Kurz
NRIC: S0000003J; Phone: 95352563; Email: heinz@example.com; Address: wall street; Date of Birth: 6 June 1996; Gender: Male
Deleting a policy : deletepolicy
Deletes the specified policy from the address book.
Format: deletepolicy INDEX
Expired items are removed on restart of application. |
Examples:
Deletes the 2nd policy in the list of policies.
# input commands:
>>> listpolicy
>>> deletepolicy 2
Expected output:
Deleted Policy: Life Insurance
Description: Insurance for life; Coverage: 50 years, 0 months, 0 days; Price: $1000000; Start Age: 21; End Age: 80
Criteria: [high blood pressure]
Tags: [term insurance]
Deletes the 1st policy in the results of the findpolicy
command.
# input commands:
>>> findpolicy senior
>>> deletepolicy 1
Expected output:
Deleted Policy: Senior Care
Description: Care for seniors; Coverage: 5 years, 11 months, 20 days; Price: $50000; Start Age: 50; End Age: 75
Restore Deleted Items: restore
Restores an item (person or policy) in the bin specified by the user.
Format:
restore INDEX
Examples:
Restores first index of the list of bin items.
# input commands
>>> listbin
>>> restore 1
Expected Output:
Restored item: David Georgia NRIC: S0000010T Phone: 94820001 Email: davegeorge@example.com Address: 10th Fly street
Date of birth: 5 March 1980 Gender: Male Date deleted: 22 Oct 2019 at 09:57 PM Expiry Date: 21 Nov 2019 at 09:57 PM
Set expiry time of bin items: binitemexpiry
User can set the amount of time bin items stay in the bin before they are permanently removed.
Format:
binitemexpiry UNIT/AMOUNT
Example:
binitemexpiry days/30
Set all BinItems to expire 30 days their deletion. They will be removed permanently once they exceed their expiry date.
Expected Output:
Changed bin item expiry time! Items in the Bin will be removed permanently 30 days after their deletion.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Bin Feature
Implementation
The bin feature is facilitated by BinItem
, UniqueBinItemList
classes and the interface Binnable
. Objects that
can be "binned" will implement the interface Binnable
. When a Binnable
object is deleted, it is wrapped in a
wrapper class BinItem
and is moved into UniqueBinItemList
.
The follow class diagram shows how bin is implemented.
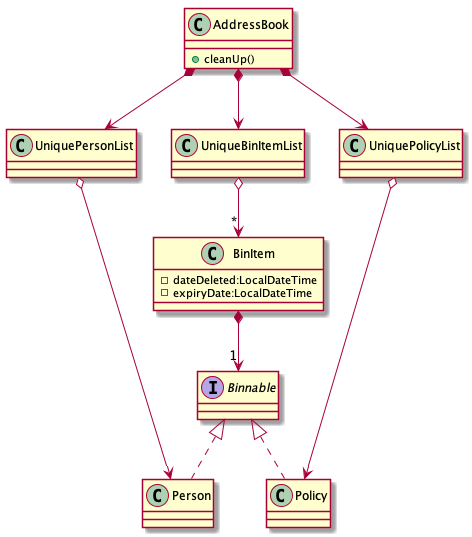
BinItem
has 2 key attributes that is wrapped on top of the Binnable
object, namely: dateDeleted
and expiryDate
.
Objects in the bin stays there for 30 days, before it is automatically deleted forever. Both attributes are used in the
auto-deletion mechanism of objects in the bin.
Given below is an example usage scenario and how the bin mechanism behaves at each step.
Step 1. When the user launches Insurelytics, ModelManager
will run ModelManager#binCleanUp()
, which will check the
expiryDate
of all objects in UniqueBinItemList
against the system clock. If the system clock exceeds expiryDate
,
UniqueBinItemList#remove()
is called and deletes the expired object forever.
Step 2. The user executes deletepolicy 1
command to delete the first policy in the address book. The deletepolicy
command calls the constructor of BinItem
with the deleted policy to create a new BinItem
object. At this
juncture, the attribute dateDeleted
is created, and expiryDate
is generated by adding TIME_TO_LIVE
to
dateDeleted
. At the same time, references to the policy that was just deleted will also be removed from any BinItem
that has them.
Removing references of deleted policies in items inside the bin only happens for deletepolicy . Removing of
references does not happen for deleted persons, since policies don’t keep track of the persons that bought them.
|
Step 3. The deletepolicy
command then calls Model#addBinItem(policyToBin)
and shifts the newly created BinItem
to
UniqueBinItemList
.
The following sequence diagram shows how a deletepolicy
operation involves the bin.
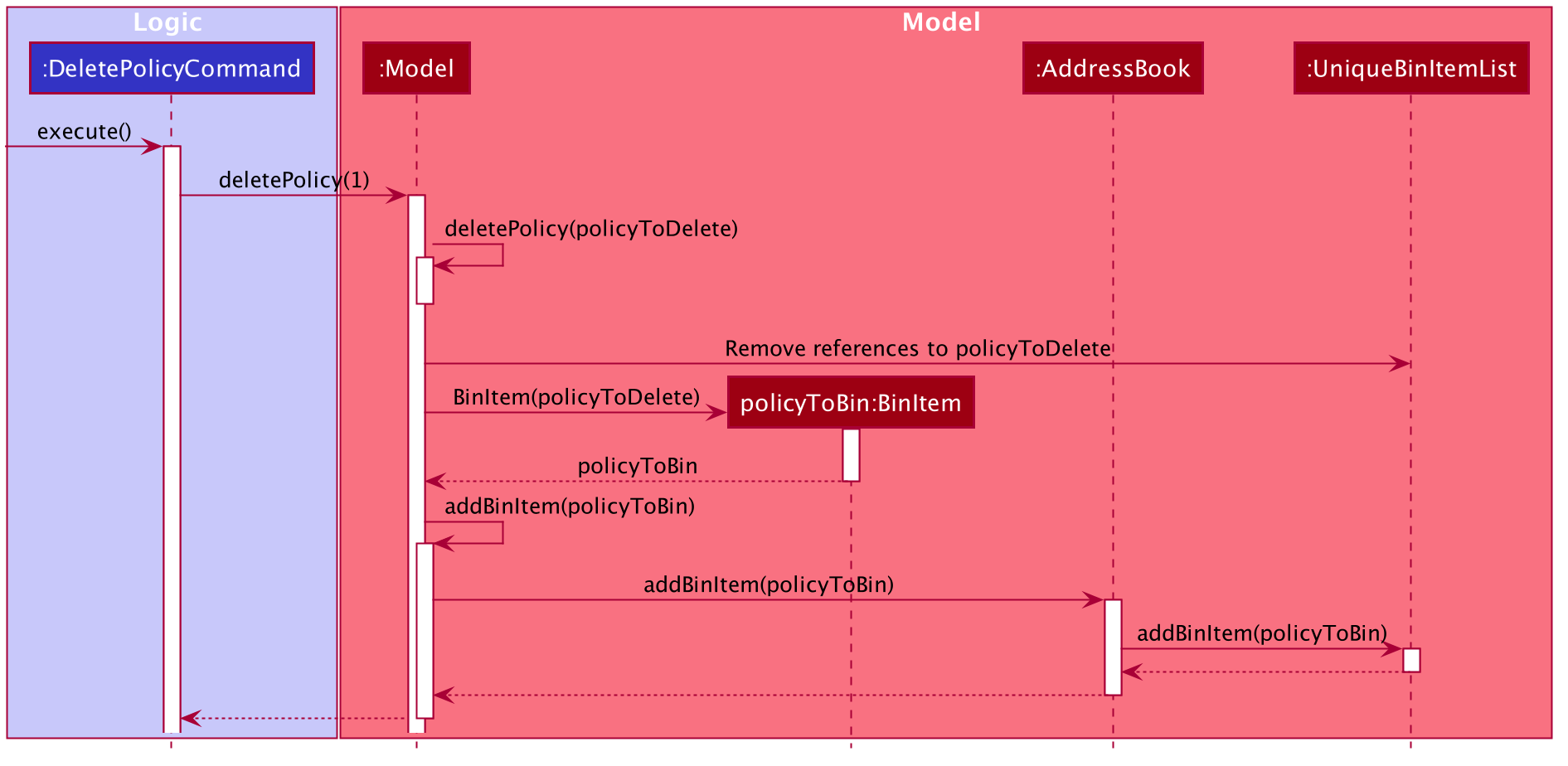
Step 4. The user quits the current session and starts a new session some time later. He/she then realises that he/she
needs the person that was deleted and wants it back, so he/she executes restore 1
to restore the deleted person
from the bin.
Step 5. The restore
command then calls Model#deleteBinItem(itemToRestore)
, which removes itemToRestore
from
UniqueBinItemList
. The wrapper class BinItem
is then stripped and the internal policy item is added back to
UniquePolicyList
.
The following sequence diagram shows how a restore command operates.
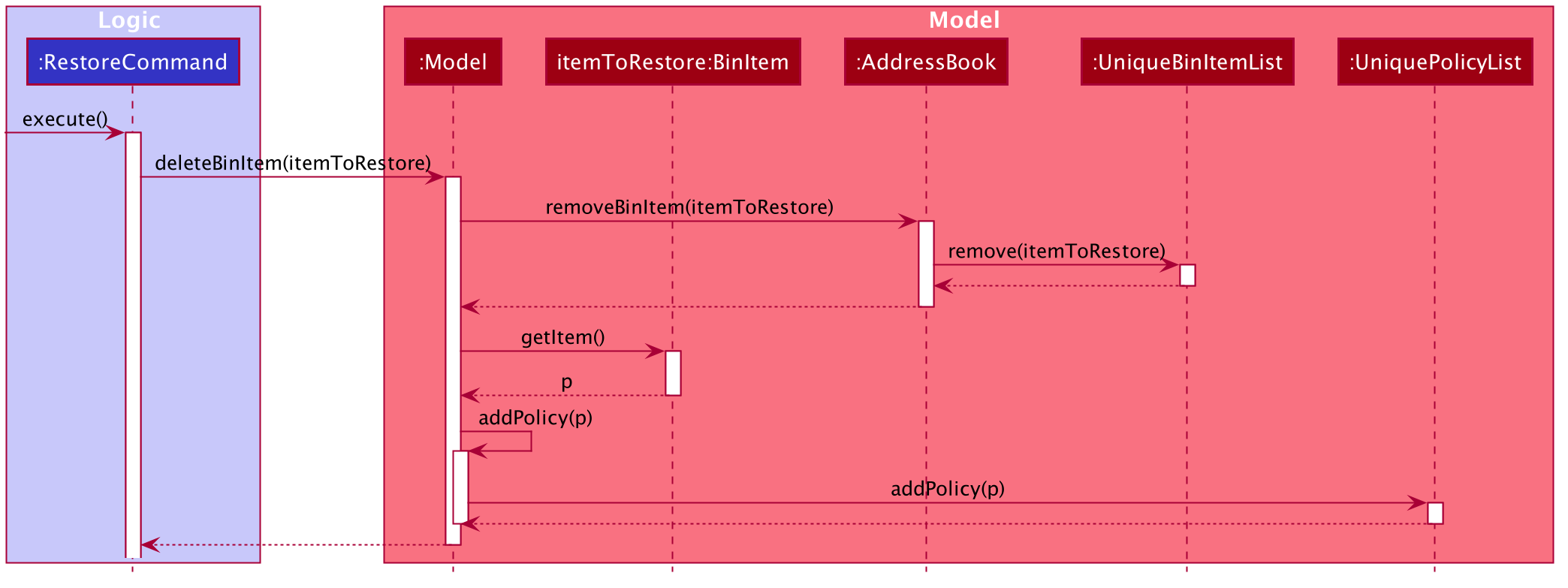
The following activity diagram summarizes the steps above.
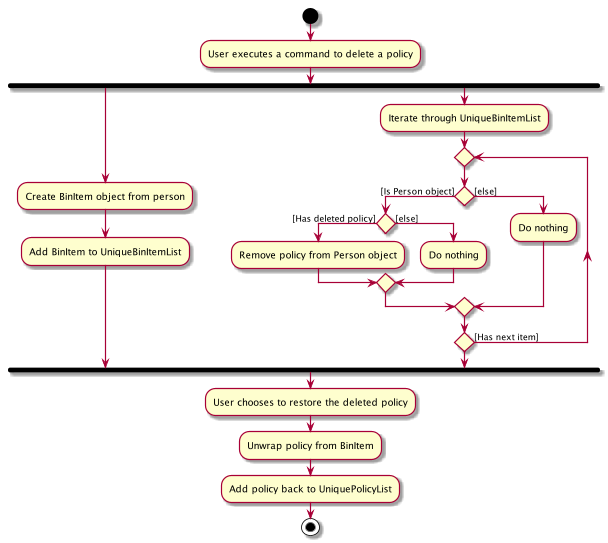
Design Considerations
Aspect: Which part of the architecture does Bin belong
-
Alternative 1 (current choice): As part of AddressBook
-
Pros: Lesser repeated code and unnecessary refactoring. Other features at the AddressBook level such as undo/redo will not be affected with a change/modification made to Bin as it is not dependent on them.
-
Cons: From a OOP design point of view, this is not the most direct way of structuring the program.
-
-
Alternative 2: Just like AddressBook, as part of Model
-
Pros: More OOP like and lesser dependencies since Bin is extracted out from AddressBook. Methods related to bin operations are called only from within Bin.
-
Cons: Many sections with repeated code since it is structurally similar to AddressBook.
-
Use case: Delete person
MSS
-
User requests to list persons.
-
Insurelytics shows a list of persons.
-
User requests to delete a specific person in the list.
-
Insurelytics deletes the person.
-
Person appears in the recycling bin.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Insurelytics shows an error message.
Use case resumes at step 2.
-
Use case: Restoring recently deleted items
MSS
-
User requests to list recently deleted items from bin.
-
Insurelytics shows a list of bin items.
-
User requests to restore a specific item in the list.
-
AddressBook restores the item to the list it belongs to.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Insurelytics shows an error message.
Use case resumes at step 2.
-